Spring Boot - Welcome Page 만들기
프로젝트 셋팅 방법 : https://jin2rang.tistory.com/entry/Spring-Boot-%EC%85%8B%ED%8C%85%ED%95%98%EA%B8%B0-startspringio 프로젝트 기초적인 셋팅을 완료한 뒤 View의 Welcome Page를 띄워보려고 한다. 프로..
jin2rang.tistory.com
Thymeleaf
Integrations galore Eclipse, IntelliJ IDEA, Spring, Play, even the up-and-coming Model-View-Controller API for Java EE 8. Write Thymeleaf in your favourite tools, using your favourite web-development framework. Check out our Ecosystem to see more integrati
www.thymeleaf.org
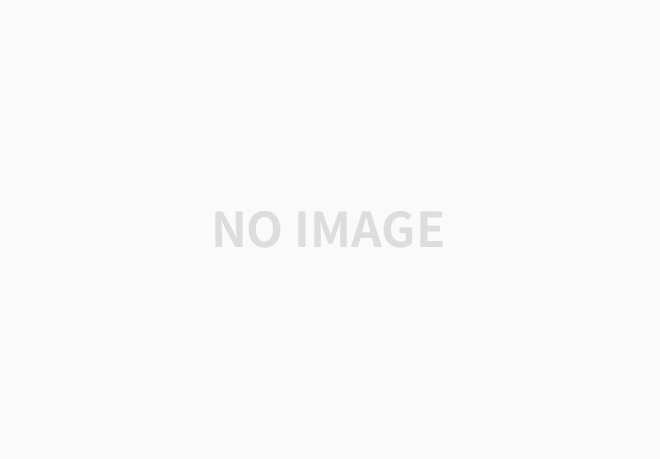
Controller에서 데이터를 전달하기 위해 Controller패키지를 생성하고 , Controller파일을 생성했다.
package com.jin2rang.hello.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model) {
model.addAttribute("data", " Here's jin2rang blog !!");
return "hello";
}
}
@GetMapping의미는 HTTP메소드 중 GET으로 요청하는 것을 의미하고, URL요청할 때 컨트롤러의 메서드와 맵핑할때 사용한다.
@GetMapping("hello")라고 작성하면 /hello라고 요청했을 때 public String hello(Model model) {...} 이 부분이 동작한다.
현재 이 서버는 localhost:8080으로 실행되기 때문에 localhost:8080/hello라고 요청하면 해당 결과를 확인 할 수 있다.
templates폴더에는 hello.html을 생성한다.
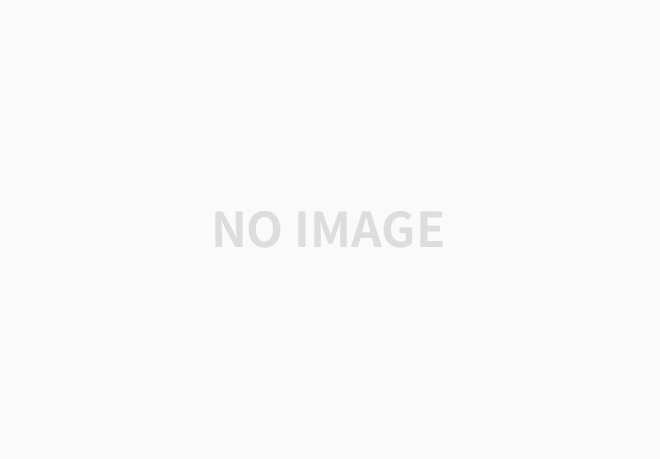
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<p th:text="'안녕하세요. ' + ${data}">반갑습니다. Welcome!!</p>
</body>
</html>
마지막으로, 위의 Controller 소스를 보면 return값이 "hello"이다.
return하는 hello는 templates폴더에 생성했던 hello.html의 이름이다.
(resources/templates/hello.html의 hello이름을 의미한다.)
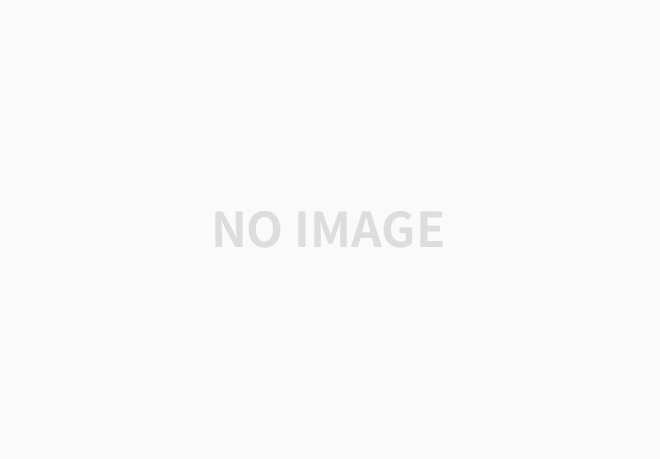
웹 브라우저에서 다음과 같이 확인했다!!
방금 실습해본 것을 정리해보면, 동작구조는 다음과 같다.
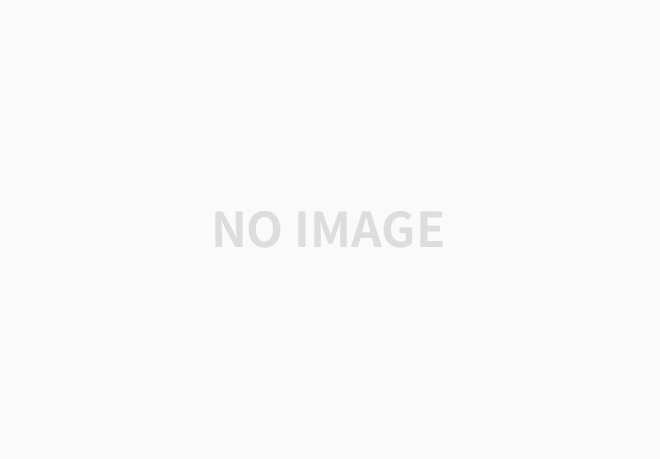
1. localhost:8080/hello로 요청
2. helloController에서 @GetMapping("hello")어노테이션이 있는 메서드와 맵핑되어 model에 data라는 key에 값을 넣어 hello와 return을 한다.
3.Spring Boot내부에 viewResolver가 resource/templates폴더에서 화면을 찾아서 처리한다.
Spring Boot 템플릿 엔진은 기본적으로 viewName을 맵핑하여 resources:templates/+{ViewName}+.html와 같이 동작한다. 그래서 hello를 return했을 때 파일을 찾아 화면을 그려주는 것이다.
4. 웹 브라우저에서 확인!
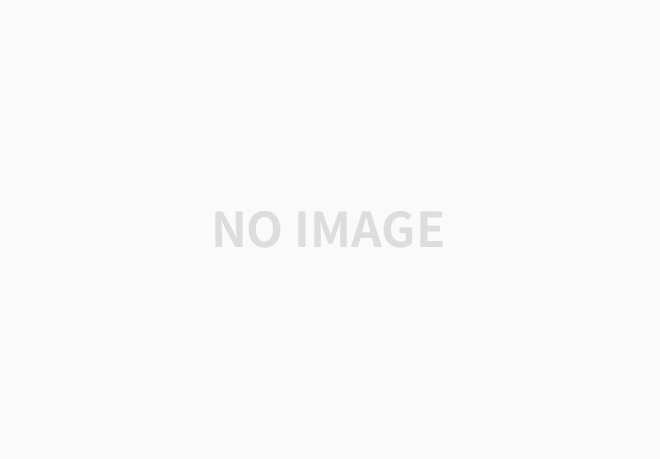
'개발 이모저모 > Spring' 카테고리의 다른 글
Spring Boot - 웹개발 기초 - ① 정적컨텐츠 (0) | 2022.06.27 |
---|---|
Spring Boot 기초 - 라이브러리 살펴보기 (0) | 2022.06.27 |
Spring Boot - Welcome Page 만들기 (0) | 2022.06.25 |
Spring Boot - Import projects (0) | 2022.06.25 |
Spring Boot 셋팅하기 - start.spring.io (0) | 2022.06.23 |